728x90
반응형
[step1] 데이터베이스 생성

※ Mysql 클라이언트 툴은 HeidSQL 사용
[step2] java 라이브러리 추가
이클립스 다이나믹 웹프로젝트를 생성하고, WEB-INF/lib 아래 다음과 같이 라이브러리 추가
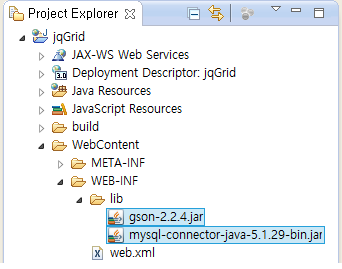
[step3] javascript 라이브러리 추가
jgGrid를 사용하기 위해서는 jQuery, jQueryUI 라이브러리가 필요하다.
다운받은 jqGrid파일의 css, js, plugins폴더를 프로젝트에 복사하고, jquery와 jqueryui관련 라이브러리도 복사하자.
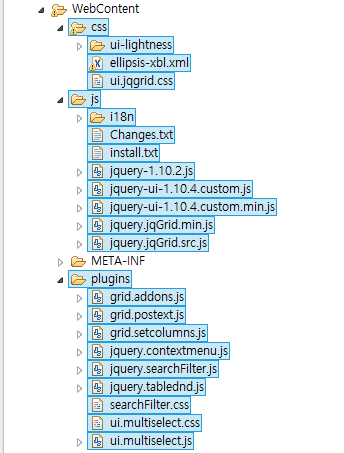
사용예)

[step4] domain class
데이터베이스의 user 테이블과 맵핑되는 클래스
-
package jqgrid.domain;
-
-
public class User {
-
private int id;
-
private int age;
-
-
public int getId() {
-
return id;
-
}
-
public void setId(int id) {
-
this.id = id;
-
}
-
return name;
-
}
-
this.name = name;
-
}
-
return email;
-
}
-
this.email = email;
-
}
-
public int getAge() {
-
return age;
-
}
-
public void setAge(int age) {
-
this.age = age;
-
}
-
}
jqGrid와 통신하기 위한 json형식의 데이트를 담을 클래스. 필드명은 동일하게 하는것이 정신건강에 도움이 될거다.^^;
-
package jqgrid.domain;
-
-
import java.util.List;
-
-
public class UserJson {
-
// 다음의 이름은 jqGrid에 정해진 이름이기에 jqGrid를 사용시 꼭 지켜야 한다.
-
private int total; // jqGrid에 표시할 전체 페이지 수
-
private int page; // 현재 페이지
-
private int records; // 전체 레코드(row)수
-
public int getTotal() {
-
return total;
-
}
-
public void setTotal(int total) {
-
this.total = total;
-
}
-
public int getPage() {
-
return page;
-
}
-
public void setPage(int page) {
-
this.page = page;
-
}
-
public int getRecords() {
-
return records;
-
}
-
public void setRecords(int records) {
-
this.records = records;
-
}
-
return rows;
-
}
-
this.rows = rows;
-
}
-
}
[step5] 목록페이지. 요청(ajax) jsp페이지
-
<%@ page language="java" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%>
-
<!DOCTYPE html>
-
<html>
-
<head>
-
<meta charset="utf-8">
-
<title>User List</title>
-
<link rel="stylesheet" type="text/css" href="css/ui-lightness/jquery-ui-1.10.4.custom.css">
-
<link rel="stylesheet" type="text/css" href="css/ui.jqgrid.css">
-
<script type="text/javascript" src="js/jquery-1.10.2.js"></script>
-
<script type="text/javascript" src="js/i18n/grid.locale-kr.js"></script>
-
<script type="text/javascript" src="js/jquery.jqGrid.min.js"></script>
-
-
<script type="text/javascript">
-
$("#user_list").jqGrid({
-
// ajax 요청주소
-
url:"/UserListAction",
-
// 요청방식
-
mtype:"post",
-
// 결과물 받을 데이터 타입
-
datatype:"json",
-
// 그리드 갭션
-
caption:"User List",
-
// 그리드 높이
-
// 그리드(페이지)마다 보여줄 행의 수 -> 매개변수이름은 "rows"로 요청된다
-
rowNum:10,
-
// rowNum변경 옵션
-
rowList:[10,15,20],
-
// 컬럼명
-
colNames:["id","name","email","age"],
-
// 컬럼 데이터(추가, 삭제, 수정이 가능하게 하려면 autoincrement컬럼을 제외한 모든 컬럼을 editable:true로 지정)
-
// edittyped은 text, password, ... input type명을 사용
-
colModel:[
-
],
-
// 네비게이션 도구를 보여줄 div요소
-
pager:"#pager",
-
autowidth:true,
-
// 전체 레코드수, 현재레코드 등을 보여줄지 유무
-
viewrecords:true,
-
// 추가, 수정, 삭제 url
-
editurl: "/UserEditAction"
-
});
-
-
// 네비게시션 도구 설정
-
$("#user_list").jqGrid(
-
"navGrid",
-
"#pager",
-
{closeAfterEdit: true, reloadAfterSubmit: true},
-
{closeAfterAdd: true, reloadAfterSubmit: true},
-
{reloadAfterSubmit: true}
-
);
-
});
-
-
</script>
-
</head>
-
<body>
-
<table id="user_list"></table>
-
<div id="pager"></div>
-
</body>
-
</html>
[step6] 목록 요청 컨트롤러(서블릿 클래스)
-
package jqgrid.contrller;
-
-
import java.io.IOException;
-
import java.io.PrintWriter;
-
import java.util.List;
-
-
import javax.servlet.ServletException;
-
import javax.servlet.annotation.WebServlet;
-
import javax.servlet.http.HttpServlet;
-
import javax.servlet.http.HttpServletRequest;
-
import javax.servlet.http.HttpServletResponse;
-
-
import com.google.gson.Gson;
-
import com.google.gson.GsonBuilder;
-
-
import jqgrid.dao.UserDao;
-
import jqgrid.domain.User;
-
import jqgrid.domain.UserJson;
-
-
@WebServlet("/UserListAction")
-
public class UserListAction extends HttpServlet {
-
private static final long serialVersionUID = 1L;
-
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
-
-
UserDao dao = new UserDao();
-
int records = dao.getCountRow();
-
-
UserJson userJson = new UserJson();
-
userJson.setTotal(total);
-
userJson.setRecords(records);
-
userJson.setPage(page);
-
userJson.setRows(list);
-
-
Gson gson = new GsonBuilder().create();
-
-
response.setContentType("application/json");
-
response.setCharacterEncoding("utf-8");
-
-
out.write(json);
-
out.flush();
-
out.close();
-
}
-
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
-
doGet(request, response);
-
}
-
-
}
[step7] 입력/수정/삭제 컨트롤러(서블릿 클래스)
-
package jqgrid.contrller;
-
-
import java.io.IOException;
-
-
import javax.servlet.ServletException;
-
import javax.servlet.annotation.WebServlet;
-
import javax.servlet.http.HttpServlet;
-
import javax.servlet.http.HttpServletRequest;
-
import javax.servlet.http.HttpServletResponse;
-
-
import jqgrid.dao.UserDao;
-
import jqgrid.domain.User;
-
-
@WebServlet("/UserEditAction")
-
public class UserEditAction extends HttpServlet {
-
private static final long serialVersionUID = 1L;
-
-
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
-
UserDao dao = new UserDao();
-
if(oper.equals("add")){
-
-
User user = new User();
-
user.setName(name);
-
user.setEmail(email);
-
user.setAge(age);
-
dao.insert(user);
-
}else if(oper.equals("edit")){
-
-
User user = new User();
-
user.setId(id);
-
user.setName(name);
-
user.setEmail(email);
-
user.setAge(age);
-
dao.update(user);
-
}else if(oper.equals("del")){
-
dao.deleteById(id);
-
}else{
-
}
-
}
-
-
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
-
doGet(request, response);
-
}
-
-
}
[step8] DAO 클래스
-
package jqgrid.dao;
-
-
import java.sql.Connection;
-
import java.sql.DriverManager;
-
import java.sql.PreparedStatement;
-
import java.sql.ResultSet;
-
import java.sql.SQLException;
-
import java.util.ArrayList;
-
import java.util.List;
-
-
import jqgrid.domain.User;
-
-
public class UserDao {
-
public void update(User user){
-
Connection conn = null;
-
PreparedStatement pstmt = null;
-
String sql = "update user set name=?,email=?,age=? where id=?";
-
try {
-
pstmt = conn.prepareStatement(sql);
-
pstmt.setString(1, user.getName());
-
pstmt.setString(2, user.getEmail());
-
pstmt.setInt(3, user.getAge());
-
pstmt.setInt(4, user.getId());
-
pstmt.executeUpdate();
-
// TODO Auto-generated catch block
-
e.printStackTrace();
-
}
-
-
}
-
-
public void insert(User user){
-
Connection conn = null;
-
PreparedStatement pstmt = null;
-
String sql = "insert into user(name,email,age) values(?,?,?)";
-
try {
-
pstmt = conn.prepareStatement(sql);
-
pstmt.setString(1, user.getName());
-
pstmt.setString(2, user.getEmail());
-
pstmt.setInt(3, user.getAge());
-
pstmt.executeUpdate();
-
e.printStackTrace();
-
}
-
} finally {
-
try {
-
pstmt.close();
-
conn.close();
-
// TODO Auto-generated catch block
-
e.printStackTrace();
-
}
-
}
-
}
-
-
-
public void deleteById(int id) {
-
Connection conn = null;
-
PreparedStatement pstmt = null;
-
String sql = "delete from user where id=?";
-
try {
-
pstmt = conn.prepareStatement(sql);
-
pstmt.setInt(1, id);
-
pstmt.executeUpdate();
-
e.printStackTrace();
-
}
-
} finally {
-
try {
-
pstmt.close();
-
conn.close();
-
// TODO Auto-generated catch block
-
e.printStackTrace();
-
}
-
}
-
}
-
-
-
// 전체 행의 수를 리턴하는 메서드
-
public int getCountRow() {
-
-
Connection conn = null;
-
PreparedStatement pstmt = null;
-
ResultSet rs = null;
-
String sql="select count(*) from user";
-
try{
-
// DB 드라이버 로딩
-
// DB 접속
-
// 쿼리 명령어 설정, 보내기, 결과물 받기
-
pstmt = conn.prepareStatement(sql);
-
rs = pstmt.executeQuery();
-
// 결과물 편집, 리턴
-
if(rs.next()){
-
return rs.getInt(1);
-
}
-
e.printStackTrace();
-
} finally {
-
// db관련 커넥션 해제
-
}
-
return 0;
-
}
-
-
// 전체 행의 데이터 리스트를 리턴하는 메서드
-
int beginRow = perPageRow * page - perPageRow;
-
Connection conn = null;
-
PreparedStatement pstmt = null;
-
ResultSet rs = null;
-
String sql="select id,name,email,age from user order by id desc limit ?,?";
-
// DB 드라이버 로딩
-
try{
-
// DB 접속
-
// 쿼리 명령어 설정, 보내기, 결과물 받기
-
pstmt = conn.prepareStatement(sql);
-
pstmt.setInt(1, beginRow);
-
pstmt.setInt(2, perPageRow);
-
rs = pstmt.executeQuery();
-
// 결과물 편집, 리턴
-
while(rs.next()){
-
User user = new User();
-
user.setId(rs.getInt("id"));
-
user.setName(rs.getString("name"));
-
user.setEmail(rs.getString("email"));
-
user.setAge(rs.getInt("age"));
-
list.add(user);
-
}
-
e.printStackTrace();
-
} finally {
-
// db관련 커넥션 해제
-
}
-
return list;
-
}
-
}
[실행화면]
리스트
추가
수정
삭제
728x90
반응형
'jqGrid > 소스코드' 카테고리의 다른 글
[jQuery] jqGrid - loadColplete 옵션을 이용한 특정 Row Color 변경하기 (0) | 2015.10.22 |
---|---|
jQgrid의 지정한 칼럼, 지정한 열을 다른색으로 지정하기 (0) | 2015.10.22 |
창크기에 따라 jqgrid width 조정하기 (0) | 2015.10.16 |
jqgrid 자주 쓰는 것들 (0) | 2015.09.24 |
Jqgrid ROW에 BOLD 적용 (0) | 2015.09.24 |
(JQuery UI Plugin) jqGrid 웹(HTML) GRID 컴포넌트 사용 방법 (0) | 2015.05.29 |
jqGrid - Step2) local에서 임시데이터로 Display Test (0) | 2015.05.29 |
jqGrid - Step1) jquery와 jqgrid Plugin의 다운로드와 include (0) | 2015.05.29 |